Editor’s note: This piece was originally published in our LinkedIn newsletter, Branching Out_. Sign up now for more career-focused content > |
Everyone’s talking about it: AI is changing how we work. And nowhere is that more true than in the field of software engineering.
If you’re just getting started as a developer, you might be wondering: is AI ruining my chances of getting a junior-level role? After all, a 2023 study by ServiceNow and Pearson projects that nearly 26% of tasks performed by [current] junior application developers will be augmented or fully automated by 2027.
In a word: No. Quite the contrary. New learners are well positioned to thrive as junior developers because they’re coming into the workforce already savvy with AI tools, which is just what companies need to adapt to the changing ways software is being developed.
Our CEO Thomas Domke says:
We’re entering an era where interns and junior developers are showing up already fluent in the best tools for AI code-gen on the market. Why? They vibe with AI. They build with it. Fresh talent ➡ better ideas ➡ the best tools. :robot_face:
— Thomas Dohmke (@ashtom) May 27, 2025
Thanks @GergelyOrosz for the story on how we’re… https://t.co/Sj6KAbq7dz
Hear more from Thomas on The Pragmatic Engineer podcast >
So what does that mean for you? According to Miles Berry, professor of computing education at the University of Roehampton, today’s learners must develop the skills to work with AI rather than worry about being replaced by it. As a junior developer, you need to think critically about the code your AI tool gives you, stay curious when things feel unfamiliar, and collaborate with AI itself in addition to senior team members.
As Berry puts it:
“Creativity and curiosity are at the heart of what sets us apart from machines.”
With that in mind, here are five ways to stand out as a junior developer in the AI era:
1. Use AI to learn faster, not just code faster
Most developers use GitHub Copilot for autocomplete. But if you’re just starting out, you can turn it into something more powerful: a coding coach.
Get Copilot to tutor you
You can set personal instructions so Copilot guides you through concepts instead of handing you full solutions. Here’s how:
In VS Code, open the Command Palette and run:
> Chat: New Instructions File
Then paste this into the new file:
---
applyTo: "**"
---
I am learning to code. You are to act as a tutor; assume I am a beginning coder. Teach me concepts and best practices, but don’t provide full solutions. Help me understand the approach, and always add: "Always check the correctness of AI-generated responses."
This will apply your tutoring instructions to any file you work on. You can manage or update your instructions anytime from the Chat > Instructions view.
Ask Copilot questions
Open Copilot Chat in VS Code and treat it like your personal coach. Ask it to explain unfamiliar concepts, walk through debugging steps, or break down tricky syntax. You can also prompt it to compare different approaches (“Should I use a for
loop or map
here?”), explain error messages, or help you write test cases to validate your logic. Every prompt is a learning opportunity and the more specific your question, the better Copilot can guide you.
Practice problem solving without autocomplete
When you’re learning to code, it can be tempting to rely on autocomplete suggestions. But turning off inline completions — at least temporarily — can help strengthen your problem-solving and critical thinking skills. You’ll still have access to Copilot Chat, so you can ask questions and get help without seeing full solutions too early.
Just keep in mind: This approach slows things down by design. It’s ideal when you’re learning new concepts, not when you’re under time pressure to build or ship something.
To disable Copilot code completion for a project (while keeping chat on), create a folder called .vscode
in the root of your project, and add a file named settings.json
with this content:
{
"github.copilot.enable": {
"*": false
}
}
This setting disables completions in your current workspace, giving you space to think through solutions before asking Copilot for help.
Read our full guide on how to use Copilot as a tutor >
2. Build public projects that showcase your skills (and your AI savvy)
In today’s AI-powered world, highlighting your AI skills can help you stand out to employers. Your side projects are your portfolio and GitHub gives you the tools to sharpen your skills, collaborate, and showcase your work. Here’s how to get started: In VS Code, open Copilot Chat and type:
/new
Copilot can scaffold a new project inside your editor to help you get started. Once it’s scaffolded, ask Copilot:
“Add the MIT license to this project and publish it as a public project on GitHub.”
Open a command line in VS Code and send the following prompt to manually push:
git init && git add . && git commit -m "Initial commit" && git push
Or create a new repo using the GitHub web interface and upload your files.
From there, you can:
- Track progress with issues, commits, and project boards.
- Document your journey and milestones in the README.
- Iterate and improve with feedback and AI assistance.
Read our full guide on prompting Copilot to create and publish new projects and start building your public portfolio >
3. Level up your developer toolkit with core GitHub workflows
Yes, AI is changing the game, but strong fundamentals still win it. If you’re aiming to level up from student to junior dev, these core workflows are your launchpad:
- Automate with GitHub Actions. Automating builds and deployments is a best practice for all developers. Use GitHub Actions to build, test, and deploy your projects automatically.
- Contribute to open source. Join the global developer community by contributing to open source. It’s one of the best ways to learn new skills, grow your resume, and build real-world experience.
- Collaborate through pull requests. Coding is a team sport. Practice the same pull request workflows used by professional teams: Review others’ code, discuss feedback, and merge with confidence.
Read our full guide on understanding GitHub workflows >
4. Sharpen your expertise by reviewing code
One of the fastest ways to grow as a developer is to learn from the reviews given by your peers. Every pull request is a chance to get feedback — not just on your code, but on how you think, communicate, and collaborate.
GitHub staff engineer Sarah Vessels has reviewed over 7,000 pull requests. She advises not to be afraid to ask questions during code reviews. If you’re unsure why a suggestion was made, speak up. If something isn’t obvious, clarify. Code review is a conversation, not a test.
Here’s how to make the most of it:
- Ask questions. Use comments to understand decisions or explore alternate approaches. It shows curiosity and builds shared knowledge.
- Look for patterns. Repeated suggestions often point to best practices you can internalize and reuse.
- Take notes. Keep track of feedback you’ve received and how you’ve addressed it, which is great for personal growth and future reference.
- Be gracious. Say thank you, follow up when you make changes, and acknowledge when a comment helped you see something differently.
Read our full guide on code review best practices and learn how to grow from every review >
5. Debug smarter and faster with AI
Debugging is one of the most time-consuming parts of software development. But with GitHub Copilot, you don’t have to do it alone.
Use Copilot Chat to:
- Ask “Why is this function throwing an error?” and get real-time explanations.
- Use
/fix
to highlight code and generate a potential fix. - Run
/tests
to create test cases and verify your logic. - Try
/explain
on cryptic errors to understand the root cause.
You can even combine commands for deeper debugging — for example, use /explain
to understand the problem, then /fix
to generate a solution, and /doc
to document it for your team.
Read our full guide on how to debug code with Copilot >
The bottom line
Whether you’re writing your first pull request or building your fifth side project, GitHub is the place to sharpen your skills, collaborate in the open, and build a portfolio that gets you hired.
AI may be reshaping the software world, but, with the right tools and mindset, junior developers can thrive.
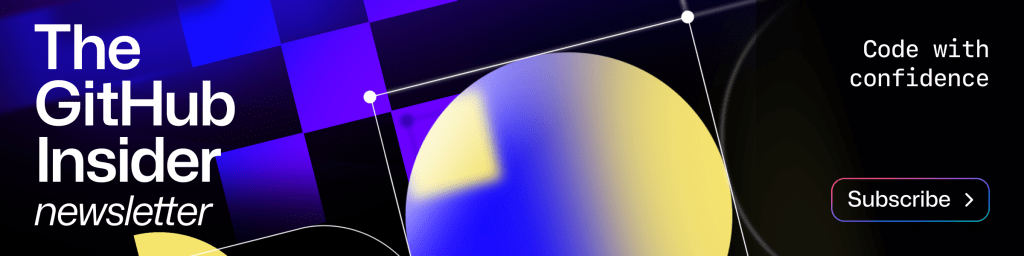
The post Junior developers aren’t obsolete: Here’s how to thrive in the age of AI appeared first on The GitHub Blog.